Kotlin Multiplatform Mobile (KMM) is a powerful framework for shared code reuse between Android and iOS applications with the assistance of Kotlin. This tutorial takes developers through the key aspects of KMM, including environment setup, shared code structure, and platform-specific API integration. With KMM, teams are able to reduce duplicated code, reduce development time, and have a single codebase for the business logic, but still enjoy native user experiences for both platforms. The tutorial also incorporates best practices, tooling support, and pitfalls to help developers implement KMM successfully in their applications.
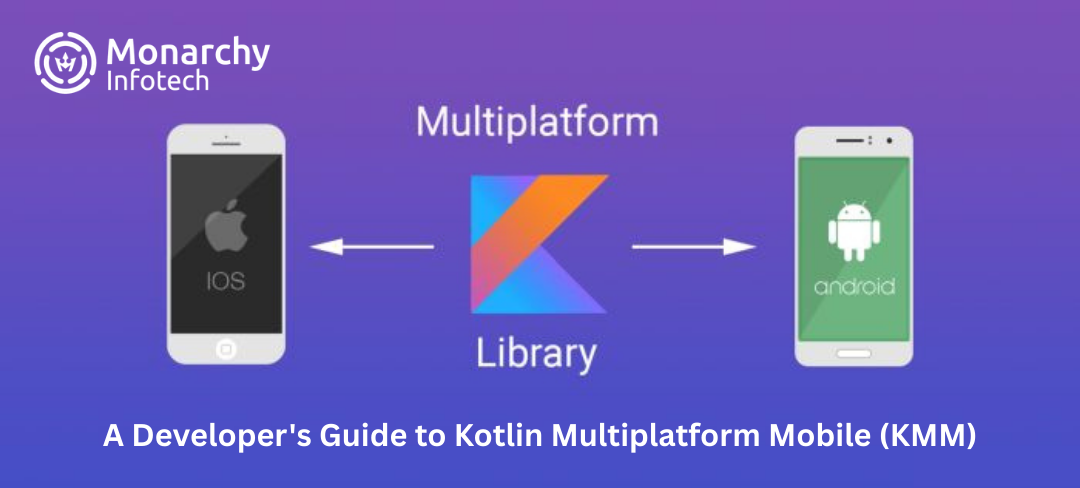
A Developer's Guide to Kotlin Multiplatform Mobile (KMM)
A Developer's Guide to Kotlin Multiplatform Mobile (KMM)
Introduction
Mobile app development usually involves developing distinct codebases for Android and iOS, which hinders development and maintenance. Kotlin Multiplatform Mobile (KMM) provides an interesting alternative by enabling developers to share business logic between platforms but still provide native experiences. In this tutorial, we will take you through the basics of KMM, demonstrating how to get started with it, how to develop shared code, and how to integrate platform-specific capabilities.
What is Kotlin Multiplatform Mobile (KMM)?
KMM is one of the components of Kotlin Multiplatform, which is a JetBrains technology that enables you to code once and execute on various platforms. KMM targets Android and iOS specifically, where core app logic like data, networking, and business rules can be shared, while UI and platform-specific APIs are native.
Why Use KMM?
Code Sharing: Create your app's fundamental logic once and reuse it on Android and iOS.
Native Performance: Tap into native APIs and create native UIs across platforms.
Faster Development: Eliminate redundant effort and accelerate delivery.
Better Maintenance: A single shared codebase makes bug fixes and feature additions easier.
Setting Up Your First KMM Project
1. Prerequisites:
- Android Studio with Kotlin plugin
- Xcode for iOS development
- JDK 11 or newer
2.Creating the Project:
- Create a new KMM project using Android Studio's Kotlin Multiplatform Mobile plugin.
- The project structure will consist of:
shared module: Kotlin code shared between Android and iOS
android App: Android-specific module
iOS App: iOS-specific module.
shared module: Kotlin code shared between Android and iOS
android App: Android-specific module
iOS App: iOS-specific module.
Understanding the Shared Module
- The shared module is written in Kotlin that compiles to both JVM bytecode (for Android) and native code (for iOS).
- Business logic, data models, repositories, and network code can be written here.
- Apply Kotlin's expect/actual mechanism where needed to implement platform-specific functionality
Writing Shared Code
Example: Simple shared logic for a greeting message
Kotlin
class Greeting {
fun greet (): String = "Hello from Kotlin Multiplatform!"
}
This class can be used directly on both platforms without modification.
Writing Shared Code
Example: Simple shared logic for a greeting message
Kotlin
class Greeting {
fun greet (): String = "Hello from Kotlin Multiplatform!"
}
This class can be used directly on both platforms without modification.
Calling Shared Code from Android and iOS
- Android: Just add the shared module as a dependency. Use shared classes as regular Kotlin code.
- iOS: Shared code gets compiled into a framework. Import it into your Xcode project and call it as any Swift or Objective-C class.